반응형
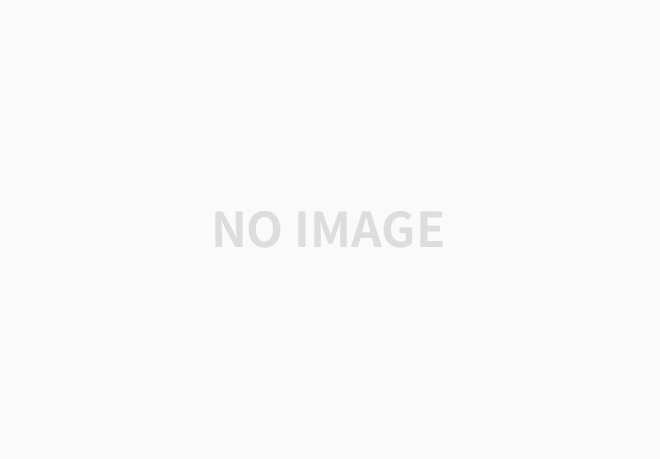
예제를 적용한 큐브들입니다. 아래 스크립트 적용시 'Z'키를 누르면 지정한 색깔 순서대로 오른쪽의 작은 큐브부터 색상이 변하게 됩니다.
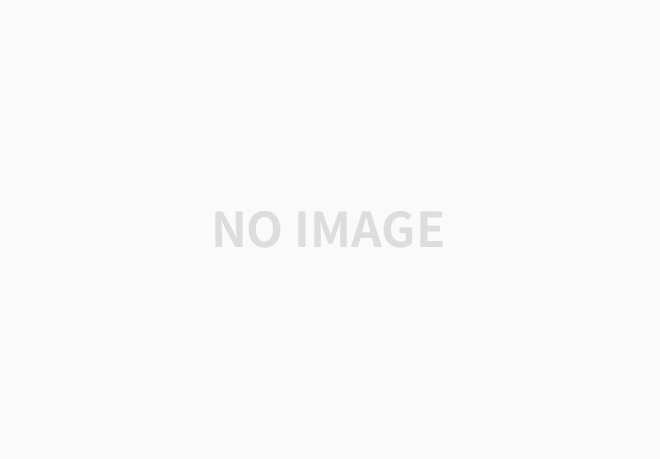
해당 큐브 그룹의 계층창과 인스펙터창입니다.
이렇게 4개의 큐브를 하나의 게임오브젝트의 자식으로 설정하고,
부모오브젝트에 스크립트를 적용하였습니다.
소스코드
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Test_CubeStream : MonoBehaviour
{
List<Color> MyColors = new List<Color>();
Dictionary<GameObject, Renderer> MyCubes = new Dictionary<GameObject, Renderer>(); //큐브들
int numberOfCube = 0;
void Start()
{
SetCubeNumber(4); // 1. 큐브수 설정
SettingColors();
for (int i = 0; i < numberOfCube; i++) // 2. 설정 수 만큼 큐브생성
MyCubes.Add( GetCube(i), GetCube(i).GetComponent<Renderer>());
}
void Update()
{
if (Input.GetKeyDown(KeyCode.Z)) // 2. Z키 입력시 색상변환
{
if (MyCubes[GetCube(0)].material.color == Color.white) // 3. 첫 입력시 첫번째 큐브 빨간색으로 변경
FirstCubeChange(MyCubes);
else
{
ChangeColor(MyCubes); // 4. 하얀색이 아닌 큐브들 색상변경 (특정 색상 로테이션)
WCubeChange(MyCubes); // 5. 순서대로 하얀색 큐브 빨간색으로 변경
}
}
}
void FirstCubeChange(Dictionary<GameObject, Renderer> MyCubes) // 3. 첫 입력시 첫번째 큐브 빨간색으로 변경
{
MyCubes[GetCube(0)].material.color = Color.red;
}
void ChangeColor(Dictionary<GameObject, Renderer> MyCubes)
{
for (int i = 0; i < MyCubes.Count; i++) // 4. 하얀색이 아닌 큐브들 색상변경 (특정 색상 로테이션)
{
for (int j = 0; j < MyColors.Count; j++)
{
if (MyCubes[GetCube(i)].material.color == MyColors[j])
{
MyCubes[GetCube(i)].material.color = MyColors[(j + 1) % MyColors.Count];
break;
}
}
}
}
void WCubeChange(Dictionary<GameObject, Renderer> MyCubes)
{
for (int i = 0; i < MyCubes.Count -1 ; i++) // 5. 순서대로 하얀색 큐브 빨간색으로 변경
{
if (MyCubes[GetCube(i)].material.color != Color.white)
{
if (MyCubes[GetCube(i + 1)].material.color == Color.white)
{
MyCubes[GetCube(i + 1)].material.color = Color.red;
return;
}
}
}
}
void SetCubeNumber(int number)
{
numberOfCube = number;
}
GameObject GetCube(int i)
{
return GameObject.Find("Cube" + i);
}
public void SettingColors()
{
MyColors.Add(Color.blue);
MyColors.Add(Color.green);
MyColors.Add(Color.yellow);
MyColors.Add(Color.gray);
MyColors.Add(Color.black);
MyColors.Add(Color.red);
}
}
반응형
'유니티 > 스크립트' 카테고리의 다른 글
유니티 Rest API 통신 UnityWebRequest (0) | 2021.07.01 |
---|---|
유니티 특정 시간동안 조건을 유지할 경우 (0) | 2020.12.25 |
[유니티] npc와 대화하기 UniRX (1) | 2020.12.24 |
[유니티]공 이동거리 계산 프로그램 (0) | 2020.08.02 |